Creating clean, readable code is a primary imperative when working with large systems. Today, I have factored the knockout view model from a single object for both projects into an inheritance based hierarchy. Now in the middle of it, I’m replacing a programmingLanguage variable with a language class that also handles the language for the style sheet (LESS, SCSS) and document (Jade, ECO).
var Language = Class.$extend({
__init__: function () {
LANGUAGE = {
PYTHON: 0,
JAVASCRIPT: 1,
LESS: 2,
CSS: 3,
HTML: 4
};
LANGUAGE_TYPE = {
STYLE: 1,
}
}
})
Then I paused and thought it didn’t seem right. I remember Enums were more terse in C. So I decided to us an Enum class.
function Enum() {
var obj = new Object();
for (var i = 0; i < arguments.length; i++) {
obj[arguments[i]] = i;
}
return obj;
}
var Language = Class.$extend({
__init__: function () {
LANGUAGE = Enum('PYTHON', 'JAVASCRIPT', 'LESS', 'CSS', 'HTML');
LANGUAGE_TYPE = Enum('SCRIPT', 'STYLE', 'DOCUMENT');
}
})
Now I like that much better. It’s like automatic numbering, except I don’t have to read it.
I just had an aha! insight 5 minutes ago when working on the design to be implemented next month. The previous storage format used string splitting and joining. Everyone who’s worked with it recognizes the mistake immediately, though the solution didn’t come until I spent a bit of time designing the system.
Previously, get_code and set_code belonged to the abstract factory. Looking at the way things are used, it may better facilitate the implementation of the local history feature if a code storage class was used. I took a snapshot when I got the idea for the JSON format, so things are still a bit disorganized:
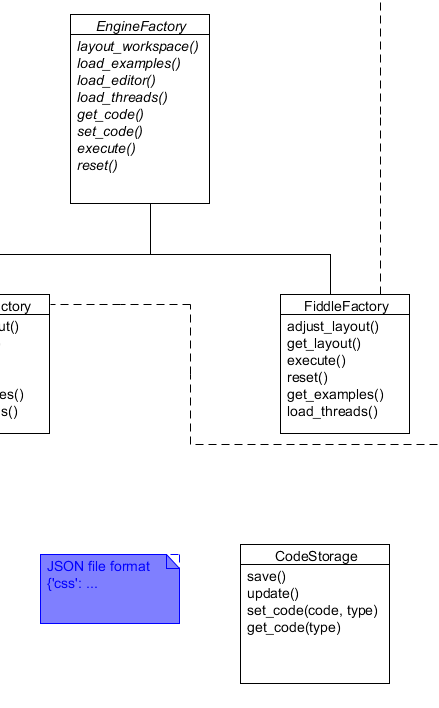
The realization is that this design doesn’t require the back-end to store all the different languages used in the fiddle. It allows both PythonFiddle, which stores only Python, and FiddleSalad, which mixes languages, to use the same storage backend. They already do, but I’m getting ready to go to the next stage. The /python/ and /coffeescript/ URL structure is still good for SEO and linking purposes.
Just working on this project yesterday, I discovered the Facebook login was no longer working. I noticed from my other projects Facebook changed their API and the library needed to be upgraded. Back in 2009, the best library available was socialauth. Now I’m using social_auth in my newer projects. There’s more documentation at first glance. Although problems showed up from time to time, upgrading always fixed them. So I would recommend social_auth.
It turns out I could use an independent login module, already a part of the shared codebase for 2 other projects, at a cost. The cost is to add the login button to keep the logic flow the same. Later, I used a dependent observable to automatically save after the authentication state changes. Simply pass the load or save function as an argument to the login decorator (Python term, same concept in JavaScript):
loginRequired: function (action) {
if (viewModel.authenticated()) {
return false;
} else {
viewModel.setMessage(loginPrompt, 'warning');
viewModel.afterLogin = action;
return true;
}
},
The save function begins by checking if the user is logged in:
save: function () {
if (viewModel.loginRequired(viewModel.save)) return;
afterLogin is called in the observable:
ko.dependentObservable(function() {
if (viewModel.authenticated() && viewModel.message() == loginPrompt) {
viewModel.setMessage('');
viewModel.afterLogin();
}
});
After a hectic few minutes of upgrading the site with about 3 different kinds of server errors and many email debug messages, I finished upgrading the database and the settings file.
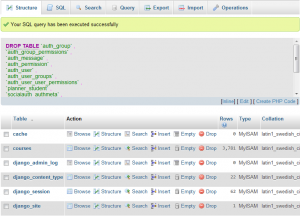
Maybe next time I will use if DEBUG statements in my settings file so it can be copied straight to server. But the main reason I opted not to was because of the facebook, twitter, and google keys which had to be set differently. On the other hand, those can be in a conditional debug statement, too.
Overall, it was a thrill to work with the project again, bring back the gifts from more recent projects, and see the brilliantly written code.
While working with the command line and SQL, I accidentally discovered a shortcut for the recycle bin on Windows. That is, the $ Recycle Bin(ary).
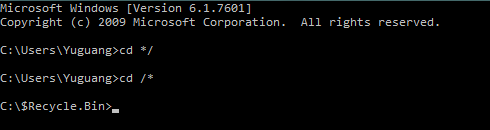
Yes, that means everything resides in the recycle bin. Literally, /* means everything under the root. All things under the root are being continually recycled. Coincidentally, I am recycling lots old code. Throwing stuff away and putting the new parts in.
The other observation is that */ is here. Everything leads here.
Compatibility with the old API means a library can be upgraded without affecting the codebase. I recently upgraded these libraries used in coursetree:
jQuery UI <1.8.14 -> 1.8.17
Raphaël 1.5 -> 2.0.2
In the case of Raphaël, the size was reduced from 151 kB to 86 kB. However, the JIT and Knockout libraries did not maintain compatibility, because I was an early adopter of the canvas and MVC libraries. Regardless, there is a speed improvement for new visitors because I combined many small JavaScript files into larger ones.